Introduction
In the realm of software development, performance is a critical aspect that can significantly impact the usability and success of an application. Java, being one of the most widely used programming languages, offers numerous tools and techniques to optimise code for speed and efficiency. This article delves into the intricacies of Java performance optimisation, exploring various strategies, best practices, and tools to help developers enhance the speed and responsiveness of their Java applications.
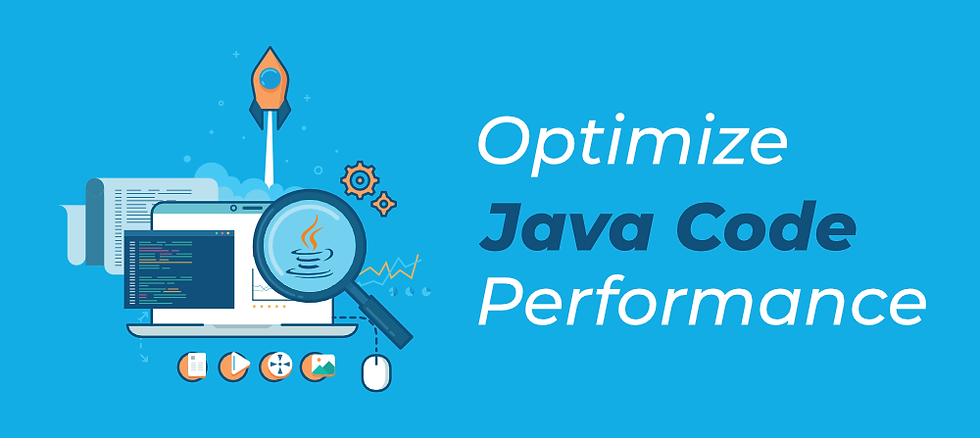
Understanding Java Performance
Before diving into optimisation techniques, it’s essential to understand the factors that influence Java performance. Java applications run on the Java Virtual Machine (JVM), which translates Java bytecode into machine code. The performance of Java applications is influenced by:
JVM Configuration: The JVM offers numerous configuration options that can significantly affect performance, such as garbage collection settings, heap size, and runtime flags.
Code Efficiency: The way code is written and structured can impact execution speed. Inefficient algorithms and data structures can lead to performance bottlenecks.
Resource Management: Efficient management of system resources like memory, CPU, and I/O can enhance application performance.
Concurrency: Proper handling of multithreading and parallel processing can improve the performance of applications that need to handle multiple tasks simultaneously.
Profiling and Benchmarking
Profiling and benchmarking are crucial steps in identifying performance issues in Java applications. Profiling involves analysing the application's runtime behaviour to pinpoint performance bottlenecks, while benchmarking measures the performance of specific code segments under various conditions.
Profiling Tools
Several tools can help profile Java applications:
VisualVM: An all-in-one tool for monitoring and troubleshooting Java applications. It provides insights into memory usage, CPU usage, thread activity, and more.
JProfiler: A robust profiling tool that offers comprehensive CPU, memory, and thread profiling, along with support for various JVMs and integrations with popular IDEs.
YourKit: A powerful profiler that provides detailed information about CPU and memory usage, allowing developers to identify and fix performance issues.
Benchmarking Techniques
Benchmarking involves measuring the performance of specific code sections to identify inefficiencies.
Key practices include:
Microbenchmarking: Testing small sections of code to measure their performance. The Java Microbenchmarking Harness (JMH) is a popular tool for this purpose.
Real-world Testing: Running the application under realistic conditions to measure its performance and identify potential bottlenecks.
Continuous Performance Testing: Integrating performance tests into the continuous integration/continuous deployment (CI/CD) pipeline to catch performance regressions early.
Code Optimisation Strategies
Optimising Java code for performance involves various strategies, ranging from algorithm optimisation to efficient resource management. Here are some key techniques:
Algorithm Optimisation
Choosing the right algorithms and data structures is crucial for performance:
Optimise Algorithms: Ensure that algorithms are efficient in terms of time and space complexity. For example, sorting algorithms like QuickSort or MergeSort are generally more efficient than BubbleSort for large datasets.
Memory Management
Efficient memory management is crucial for optimal Java performance:
Minimise Object Creation: Frequent creation and destruction of objects can lead to high garbage collection overhead. Reuse objects where possible, and consider using object pools for frequently used objects.
Optimise Garbage Collection: Configure the JVM’s garbage collection (GC) settings to suit your application's needs. The choice of GC algorithm (e.g., Serial, Parallel, CMS, G1) can have a significant impact on performance.
Avoid Memory Leaks: Ensure that objects are properly dereferenced when no longer needed to avoid memory leaks. Tools like VisualVM and YourKit can help identify memory leaks.
Efficient Use of Collections
Java’s collection framework provides various data structures that can be used efficiently to enhance performance:
Choose the Right Collection: Different collections have different performance characteristics. For example, HashMap provides constant-time complexity for get and put operations, while TreeMap guarantees logarithmic time complexity.
Use Bulk Operations: Where possible, use bulk operations like addAll, removeAll, and retainAll to minimise the overhead of multiple individual operations.
Stream API: Use the Stream API introduced in Java 8 for parallel processing and more readable code. However, be mindful of the overhead associated with creating streams.
Concurrency and Parallelism
Java provides robust support for concurrency and parallelism, which can be leveraged to enhance performance:
Thread Management: Use the ExecutorService framework to manage threads efficiently. Avoid creating and destroying threads frequently; instead, reuse threads from a thread pool.
Locking Mechanisms: Minimise the use of synchronised blocks to reduce contention. Consider using modern concurrency utilities like ReentrantLock, ReadWriteLock, and Atomic classes from the java.util.concurrent package.
Parallel Streams: Leverage parallel streams for data processing. However, ensure that the overhead of parallel processing does not outweigh the benefits for small datasets.
I/O Optimisation
Efficient handling of input and output operations can significantly improve application performance:
Buffered I/O: Use buffered streams (e.g., BufferedInputStream, BufferedReader) to reduce the overhead of I/O operations by minimising the number of system calls.
NIO (New I/O): Java’s NIO package provides non-blocking I/O operations, which can be more efficient than traditional I/O in certain scenarios.
Asynchronous I/O: Consider using asynchronous I/O for high-performance networking applications. The java.nio.channels package provides support for non-blocking sockets.
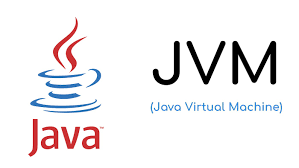
JVM Optimisation
Configuring the JVM appropriately can have a substantial impact on performance:
Heap Size: Adjust the heap size (-Xms and -Xmx options) to ensure that the JVM has enough memory to operate efficiently without triggering excessive garbage collection.
Garbage Collection Tuning: Choose an appropriate garbage collection algorithm and configure its parameters (e.g., -XX:+UseG1GC, -XX:MaxGCPauseMillis) to balance throughput and latency.
JVM Flags: Use JVM flags to enable or disable specific features. For example, -XX:+UseCompressedOops can reduce the memory footprint of 64-bit JVMs by using 32-bit object pointers.
Best Practices for Java Performance Optimisation
Following best practices can help ensure that Java applications are optimised for performance:
Code Reviews: Regular code reviews can help identify potential performance issues and ensure that best practices are followed.
Performance Testing: Integrate performance testing into the development lifecycle to catch performance issues early.
Continuous Monitoring: Monitor the performance of applications in production to identify and address performance issues promptly.
Documentation: Document performance-related decisions and configurations to ensure that they are understood and maintained by the team.
Conclusion
Optimising Java code for speed involves a combination of profiling, efficient coding practices, proper resource management, and appropriate JVM configuration. By understanding the factors that influence performance and employing the techniques discussed in this article, developers can significantly enhance the speed and responsiveness of their Java applications. Remember that performance optimisation is an ongoing process that requires continuous monitoring, testing, and refinement to keep applications running smoothly and efficiently. Enrol now our Online Java Course in Gurgaon, Kanpur, Dehradun, Kolkata, Agra, Delhi, Noida and all cities in India.
Comments