Java Multithreading: Best Practices and Pitfalls
- ashik imam
- Mar 20, 2024
- 3 min read
Introduction:
Java multithreading is a powerful feature that enables concurrent execution of tasks within a Java application. Leveraging multithreading effectively can significantly enhance the performance and responsiveness of your Java programs. However, with great power comes great responsibility. Inadequate understanding and misuse of multithreading can lead to various issues such as race conditions, deadlocks, and performance bottlenecks. In this comprehensive guide, we'll delve into the best practices for Java multithreading and highlight common pitfalls to avoid.
Understanding Java Multithreading:
Before diving into best practices and pitfalls, let's briefly review the fundamentals of Java multithreading. In Java, a thread is a lightweight process that can execute independently, allowing multiple tasks to run concurrently within a single program. Threads can be created by extending the Thread class or implementing the Runnable interface.
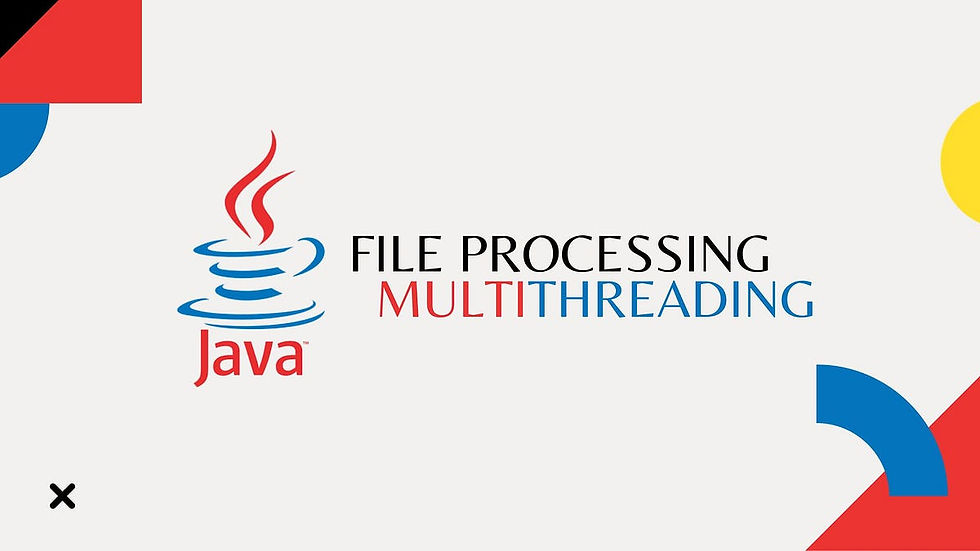
Best Practices for Java Multithreading:
Use Thread Pools: Creating a new thread for each task can be inefficient and resource-intensive. Instead, utilize thread pools provided by the Executor framework to manage and reuse threads efficiently. This approach helps in controlling the number of concurrent threads and prevents resource exhaustion.
Synchronization: Proper synchronization is crucial for ensuring thread safety and preventing race conditions. Use synchronized blocks or methods to protect shared resources from concurrent access. Additionally, consider using higher-level concurrency utilities such as java.util.concurrent.locks for more fine-grained control over locking mechanisms.
Immutable Objects: Immutable objects are inherently thread-safe since their state cannot be modified after construction. Design your classes to be immutable whenever possible to avoid synchronization issues and simplify multithreaded programming.
Concurrency Utilities: Java provides a rich set of concurrency utilities in the java.util.concurrent package. Familiarize yourself with classes such as ThreadPoolExecutor, ConcurrentHashMap, and CountDownLatch to leverage advanced concurrency features and patterns effectively.
Avoid Busy Waiting: Busy waiting, also known as spinning, involves repeatedly checking a condition within a loop until it becomes true. This approach wastes CPU cycles and can degrade performance. Instead, use synchronization primitives such as wait() and notify() or higher-level constructs like BlockingQueue for efficient inter-thread communication.
Exception Handling: Always handle exceptions gracefully within your multithreaded applications. Uncaught exceptions in threads can lead to unexpected termination and obscure debugging. Consider using Thread.UncaughtExceptionHandler to handle exceptions at the thread level and ensure proper error logging and recovery strategies.
Common Pitfalls to Avoid:
Deadlocks: A deadlock occurs when two or more threads are blocked indefinitely, waiting for each other to release resources. To prevent deadlocks, ensure that threads acquire locks in a consistent order and avoid holding multiple locks simultaneously whenever possible. Use tools like thread dump analysis and profiling to diagnose and resolve deadlock issues.
Race Conditions: Race conditions occur when the outcome of a program depends on the timing or interleaving of multiple threads. To mitigate race conditions, synchronize access to shared variables and use atomic operations or thread-safe data structures where applicable. Thoroughly review your code for potential race conditions during development and testing phases.
Performance Bottlenecks: Multithreading can improve performance by parallelizing tasks, but it can also introduce overhead due to context switching and synchronization. Profile your application using profiling tools like VisualVM or YourKit to identify performance bottlenecks and optimize critical sections of code. Consider employing techniques such as lock-free algorithms and parallel stream processing to maximize throughput.
Thread Starvation: Thread starvation occurs when one or more threads are consistently denied access to shared resources, leading to decreased throughput and responsiveness. Avoid long-running or blocking operations within synchronized blocks and periodically release locks to allow waiting threads to make progress. Monitor thread pool metrics and adjust configuration parameters accordingly to prevent thread starvation.
Memory Leaks: Improper management of thread-local resources can result in memory leaks and resource exhaustion over time. Always release acquired resources explicitly, especially in long-lived applications or environments with dynamic class loading. Use tools like Java Flight Recorder and Heap Dump Analysis to detect and diagnose memory leaks caused by multithreading.
Conclusion:
Java multithreading offers a powerful mechanism for achieving parallelism and concurrency within Java applications. By following best practices and avoiding common pitfalls, you can harness the full potential of multithreading while ensuring the reliability, performance, and scalability of your software. Remember to prioritize clarity, simplicity, and correctness in your multithreaded designs, and leverage the rich set of concurrency utilities provided by the Java platform to build robust and efficient multithreaded applications. With careful planning, testing, and continuous improvement, you can master the art of Java multithreading and unlock new possibilities for your projects. Enroll now in our Java Course Provider in Kanpur, Dehradun, Kolkata, Agra, Delhi, Noida and all cities in India to deepen your understanding of multithreading and advance your Java programming skills.
Commenti